Chart | ASP.NET - C# | ASP.NET - VB | ASP | PHP | JSP |
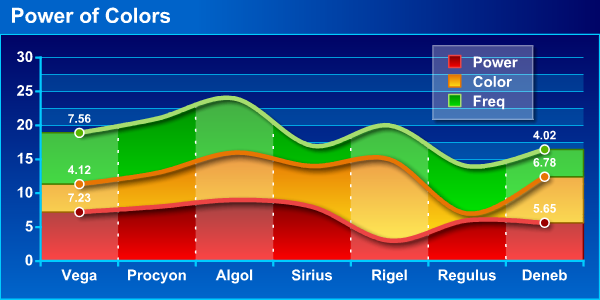
using System; using System.Data; using System.Configuration; using System.Collections; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls; using GlobFX.SwiffChartGenerator; public partial class SwiffChartGeneratorSample : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { // Set chart categories chart.SetCategoriesFromArray( new string[] { "Vega", "Procyon", "Algol", "Sirius", "Rigel", "Regulus", "Deneb" } ); // Fill the first series named "Power" chart.SetSeriesCaption( 0, "Power" ); chart.SetSeriesValuesFromArray( 0, new double[] { 7.23, 8, 9, 8, 3, 6, 5.65 } ); // Fill the second series named "Color" chart.SetSeriesCaption( 1, "Color" ); chart.SetSeriesValuesFromArray( 1, new double[] { 4.12, 5, 7, 6, 12, 1, 6.78 } ); // Fill the third series named "Freq" chart.SetSeriesCaption( 2, "Freq" ); chart.SetSeriesValuesFromArray( 2, new double[] { 7.56, 8, 8, 3, 5, 7, 4.02 } ); // Set the chart title chart.Title= "Power of Colors"; // Apply a style (*.scs) designed with Swiff Chart // The chart type is stored in the style file chart.LoadStyle( Server.MapPath("area1.scs") ); chart.OutputFormat= OutputFormat.Png; } }
Imports GlobFX.SwiffChartGenerator Partial Class SwiffChartGeneratorSample Inherits System.Web.UI.Page Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load ' Set chart categories chart.SetCategoriesFromArray(New String() {"Vega", "Procyon", "Algol", "Sirius", "Rigel", "Regulus", "Deneb"}) ' Fill the first series named "Power" chart.SetSeriesCaption(0, "Power") chart.SetSeriesValuesFromArray(0, New Double() {7.23, 8, 9, 8, 3, 6, 5.65}) ' Fill the second series named "Color" chart.SetSeriesCaption(1, "Color") chart.SetSeriesValuesFromArray(1, New Double() {4.12, 5, 7, 6, 12, 1, 6.78}) ' Fill the third series named "Freq" chart.SetSeriesCaption(2, "Freq") chart.SetSeriesValuesFromArray(2, New Double() {7.56, 8, 8, 3, 5, 7, 4.02}) ' Set the chart title chart.Title = "Power of Colors" ' Apply a style (*.scs) designed with Swiff Chart ' The chart type is stored in the style file chart.LoadStyle(Server.MapPath("area1.scs")) chart.OutputFormat = OutputFormat.Png End Sub End Class
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Swiff Chart Generator Sample</title> </head> <body> <% @Language=VBScript %> <% Response.Expires= 0 Dim chart Set chart= Server.CreateObject("SwiffChartObject.ChartObj") ' Fill the categories chart.SetCategoriesFromString "Vega;Procyon;Algol;Sirius;Rigel;Regulus;Deneb" ' Fill the first series named "Power" chart.SetSeriesCaption 0, "Power" chart.SetSeriesValuesFromString 0, "7.23;8;9;8;3;6;5.65" ' Fill the second series named "Color" chart.SetSeriesCaption 1, "Color" chart.SetSeriesValuesFromString 1, "4.12;5;7;6;12;1;6.78" ' Fill the third series named "Freq" chart.SetSeriesCaption 2, "Freq" chart.SetSeriesValuesFromString 2, "7.56;8;8;3;5;7;4.02" ' Set the chart title chart.SetTitle "Power of Colors" ' Apply a style (*.scs) designed with Swiff Chart ' The chart type is stored in the style file chart.LoadStyle Server.MapPath("area1.scs") chart.SetOutputFormat "png" Response.Write chart.GetHTMLTag Set chart= Nothing %> </body> </html>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Swiff Chart Generator Sample</title> </head> <body> <?php $install_dir= "/usr/local/SwiffChart"; require("$install_dir/resources/php/SwiffChart.php"); $chart= new SwiffChart($install_dir); // Set chart categories $chart->SetCategoriesFromArray( array( "Vega", "Procyon", "Algol", "Sirius", "Rigel", "Regulus", "Deneb" ) ); // Fill the first series named "Power" $chart->SetSeriesCaption( 0, "Power" ); $chart->SetSeriesValuesFromArray( 0, array( 7.23, 8, 9, 8, 3, 6, 5.65 ) ); // Fill the second series named "Color" $chart->SetSeriesCaption( 1, "Color" ); $chart->SetSeriesValuesFromArray( 1, array( 4.12, 5, 7, 6, 12, 1, 6.78 ) ); // Fill the third series named "Color" $chart->SetSeriesCaption( 2, "Color" ); $chart->SetSeriesValuesFromArray( 2, array( 7.56, 8, 8, 3, 5, 7, 4.02 ) ); // Set the chart title $chart->SetTitle( "Power of Colors" ); // Apply a style (*.scs) designed with Swiff Chart // The chart type is stored in the style file $chart->LoadStyle( "area1.scs" ); $chart->SetOutputFormat( "png" ); print $chart->GetHTMLTag(); ?> </body> </html>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Swiff Chart Generator Sample</title> </head> <body> <%@page language="java"%> <%@page import="com.globfx.swiffchart.SwiffChart" %> <% String install_dir= "/usr/local/SwiffChart"; SwiffChart chart= new SwiffChart(install_dir); chart.SetDocumentRoot( getServletContext().getRealPath("/") ); chart.SetAppRootUrl(request.getContextPath()); chart.SetServletInfo(request,response); // Set chart categories chart.SetCategoriesFromArray( new String[] { "Vega", "Procyon", "Algol", "Sirius", "Rigel", "Regulus", "Deneb" } ); // Fill the first series named "Power" chart.SetSeriesCaption( 0, "Power" ); chart.SetSeriesValuesFromArray( 0, new double[] { 7.23, 8, 9, 8, 3, 6, 5.65 } ); // Fill the second series named "Color" chart.SetSeriesCaption( 1, "Color" ); chart.SetSeriesValuesFromArray( 1, new double[] { 4.12, 5, 7, 6, 12, 1, 6.78 } ); // Fill the third series named "Color" chart.SetSeriesCaption( 2, "Color" ); chart.SetSeriesValuesFromArray( 2, new double[] { 7.56, 8, 8, 3, 5, 7, 4.02 } ); // Set the chart title chart.SetTitle( "Power of Colors" ); // Apply a style (*.scs) designed with Swiff Chart // The chart type is stored in the style file chart.LoadStyle( getServletContext().getRealPath("area1.scs") ); chart.SetOutputFormat( "png" ); out.println( chart.GetHTMLTag() ); chart.Release(); %> </body> </html>